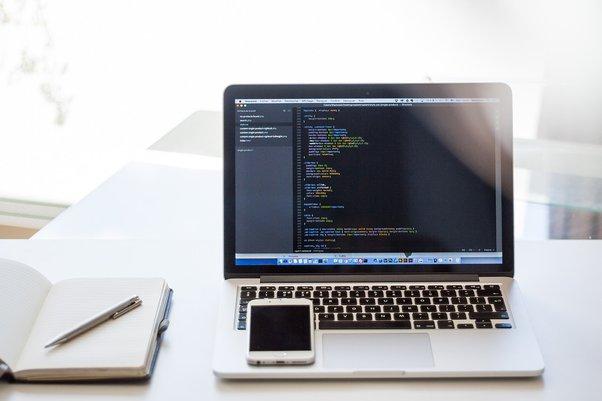
Introduction
Java, a programming language crafted by James Gosling and Mike Sheridan in the mid-1990s, stands tall as a versatile and widely-used language. It serves as the bedrock for an array of applications across diverse industries. Navigating the intricacies of core Java concepts is essential for developers seeking mastery in this language and aiming to construct resilient, scalable software. This guide embarks on an exhaustive journey through the fundamental elements that constitute the essence of Java programming.
Table of Contents
Table of Contents
- Introduction to Java
- Variables and Data Types
- Control Flow Statements
- Object-Oriented Programming (OOP)
- Exception Handling
- Multithreading
- Collections Framework
- Input/Output (I/O) Operations
- Java Database Connectivity (JDBC)
- Lambda Expressions
- Java Virtual Machine (JVM)
- Java API Documentation
Introduction to Java
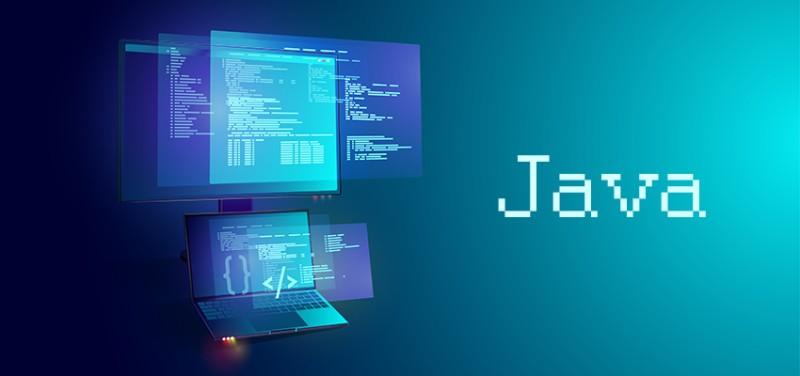
Java’s inception marked a paradigm shift in the world of programming languages. Designed with the “Write Once, Run Anywhere” (WORA) principle in mind, Java provides a platform-independent environment. This characteristic, coupled with its robustness, makes it a preferred choice for developers worldwide.
Variables and Data Types
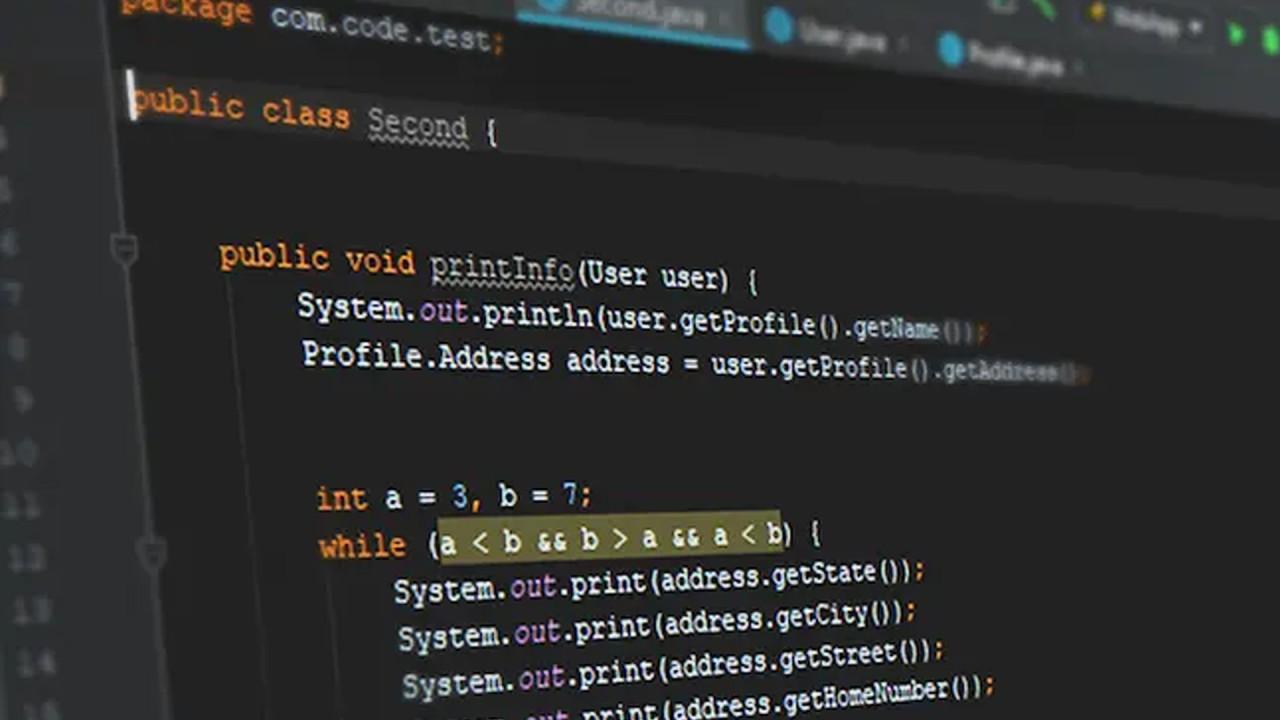
In the realm of Java, variables act as containers for storing data values. The significance of understanding data types lies in their ability to define the kind of data a variable can hold. From int and float to double and String, proper utilization of variables and data types ensures both efficient memory usage and heightened code readability.
Control Flow Statements
Control flow statements, including if-else constructs, switch statements, and loops, serve as the navigators of a Java program’s execution flow. A nuanced grasp of these statements empowers developers to steer program behavior based on conditions and iterate through data with finesse.
Object-Oriented Programming (OOP)
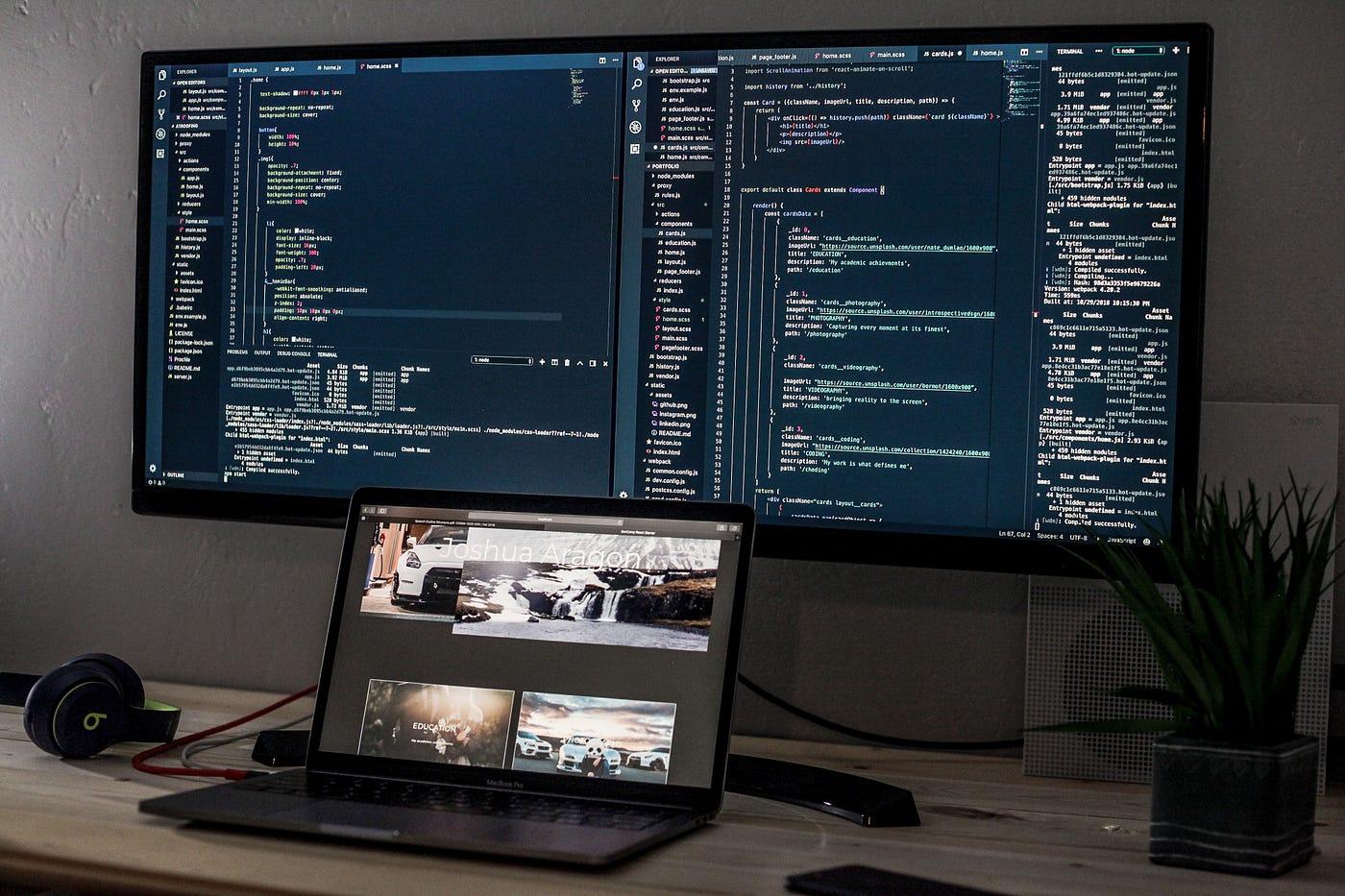
At the heart of Java lies the paradigm of Object-Oriented Programming (OOP). Concepts such as classes, objects, inheritance, polymorphism, encapsulation, and abstraction form the building blocks of Java’s OOP paradigm. Embracing these principles elevates code organization, enhances reusability, and promotes modular development.
Exception Handling
Java’s robust exception handling mechanism is a safeguard against unforeseen errors. The try-catch blocks allow developers to identify and address potential issues, preventing the abrupt termination of a program and ensuring a graceful recovery.
Multithreading
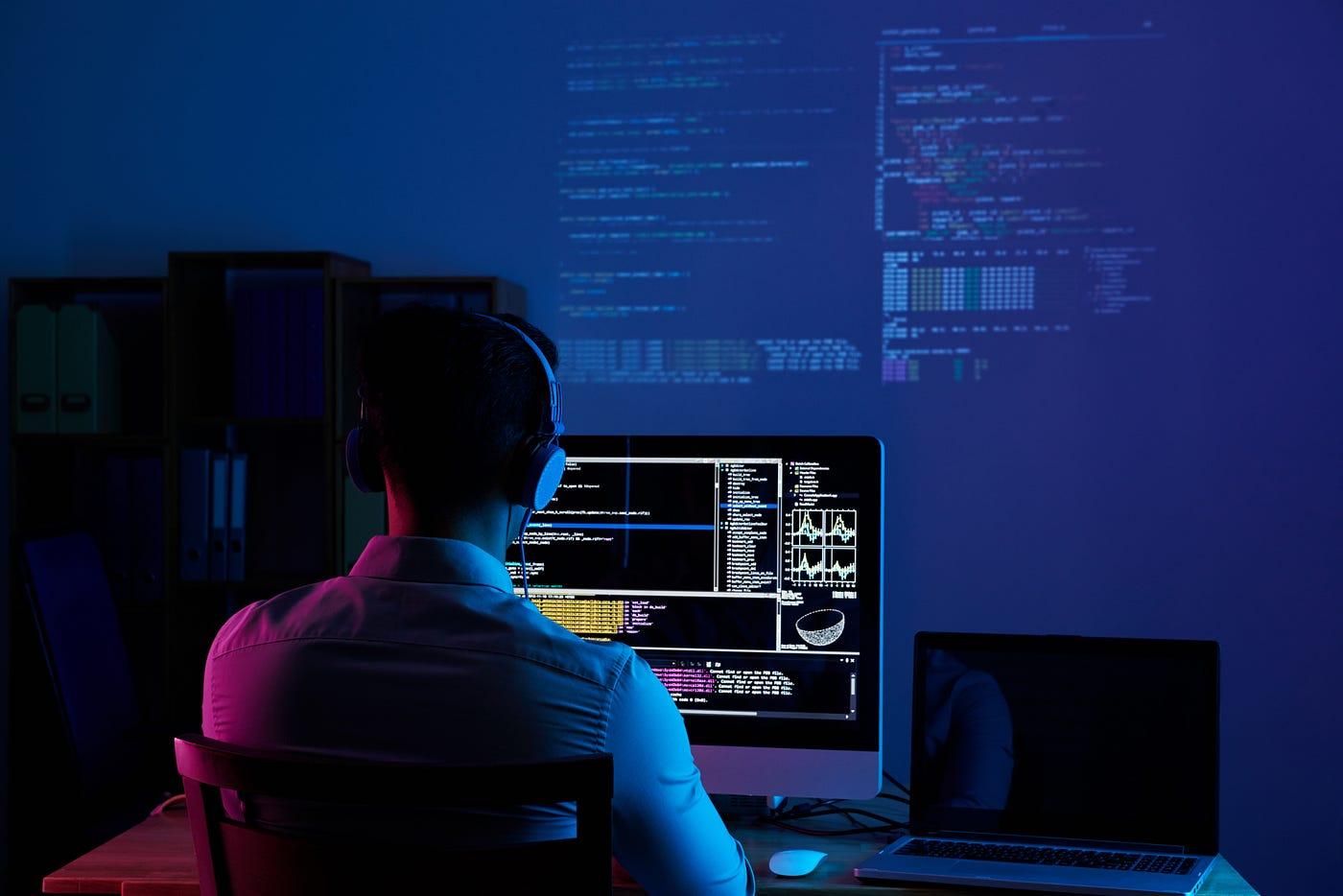
Multithreading in Java facilitates the concurrent execution of tasks, thereby enhancing program performance. The threading capabilities empower developers to create responsive applications by managing multiple threads simultaneously, harnessing the full potential of modern computing systems.
Collections Framework
The Collections Framework furnishes a comprehensive set of interfaces and classes for effective handling and manipulation of collections of objects. A solid understanding of collections, encompassing lists, sets, and maps, is imperative for efficient data management.
Input/Output (I/O) Operations
Java’s Input/Output operations form the bridge between programs and external devices. Mastery in file handling, stream processing, and serialization is indispensable for harnessing Java’s I/O capabilities to their fullest extent.
Java Database Connectivity (JDBC)
JDBC, or Java Database Connectivity, empowers Java applications to interact seamlessly with relational databases. Proficiency in JDBC is a requisite for developers building applications that necessitate data storage and retrieval from databases like MySQL, Oracle, or PostgreSQL.
Lambda Expressions
Java 8 introduced lambda expressions, offering a concise means to express functionality. Adoption of lambda expressions enhances code readability and encourages a more functional programming style.
Java Virtual Machine (JVM)
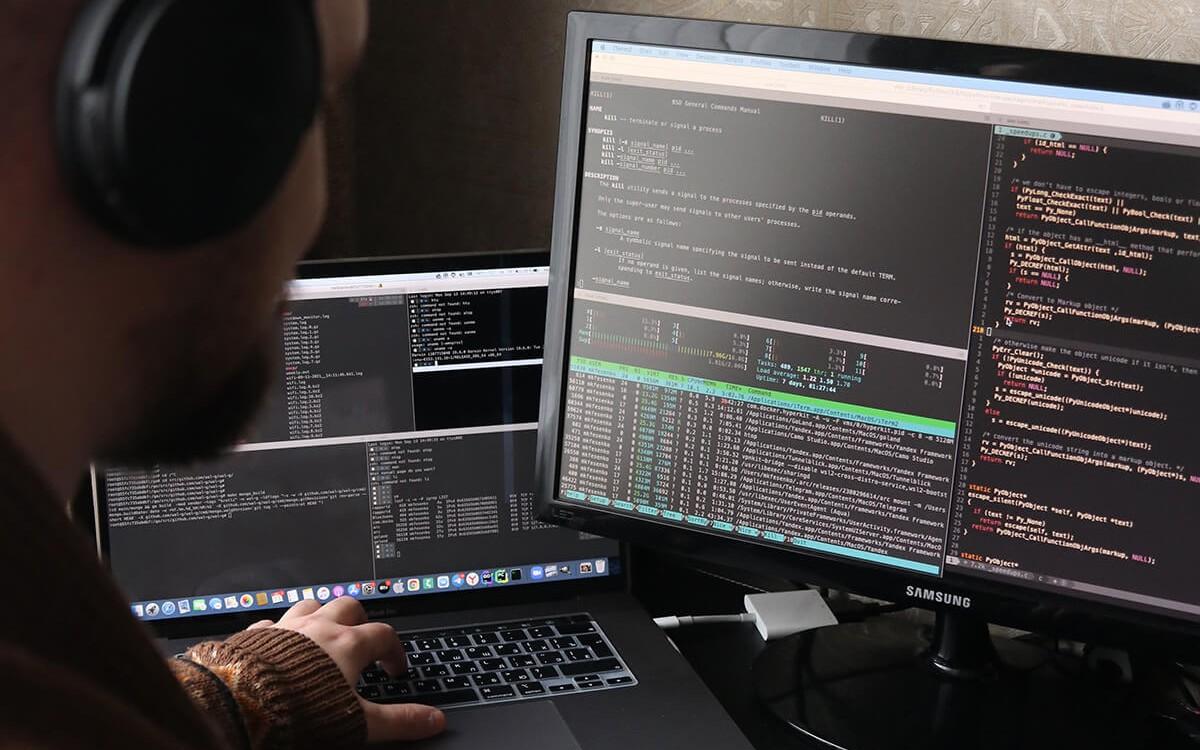
The Java Virtual Machine (JVM) is the linchpin that executes Java bytecode. A comprehensive understanding of its functioning is imperative for optimizing code performance and ensuring compatibility across diverse platforms.
Java API Documentation
The Java API documentation serves as an invaluable reference for Java’s standard library. Navigating and leveraging the documentation is a skill every Java developer should possess, aiding in efficient and effective coding.
Useful Resources
For a deeper dive into core Java concepts, explore these invaluable resources:
- Oracle’s Java Tutorials
- Oracle’s official tutorials provide in-depth explanations and examples, serving as an indispensable resource for mastering Java concepts.
- Java Programming and Software Engineering Fundamentals (Coursera)
- This Coursera specialization offers a structured learning path, encompassing Java programming and software engineering fundamentals.
FAQs (Frequently Asked Questions)
Q: What makes Java a popular programming language?
A: Java’s portability, platform independence, and robust community support contribute to its popularity. It is extensively used in web development, mobile app development, and enterprise applications.
Q: How does Java support multithreading?
A: Java’s java.lang.Thread
class and the java.util.concurrent
package provide robust support for multithreading. Developers can create and manage threads, ensuring efficient utilization of system resources.
Q: Why is exception handling important in Java?
A: Exception handling prevents unexpected errors from crashing a program. It allows developers to gracefully handle errors, improving the overall reliability and stability of Java applications.
Q: What are the key benefits of using lambda expressions in Java?
A: Lambda expressions in Java provide a concise syntax for writing anonymous methods, promoting more readable and maintainable code. They are particularly beneficial in functional programming constructs.